How to Use MCP to Let Your AI IDE See and Fix Browser Console Errors (with Cursor)
Intro
MCP (Model Context Protocol) is an open standard developed by Anthropic that allows AI agents to interact with external tools.
By giving the AI access to real-world context, MCP reduces hallucinations and expands what agents can actually do — from querying live APIs to inspecting browser state.
Why Browser Console Logs Are the Ideal First MCP Tool
One of the simplest and most effective use cases is letting the AI see your browser console logs. For web development, this is a huge unlock. Normally, if the AI builds something buggy, it's completely blind to runtime errors unless you manually copy and paste the error into your chat window.
That's not just tedious — it also breaks multi-step agent workflows. If an AI is trying to implement a feature but hits a runtime error, it often can't recover without visibility into what went wrong.
Connecting console logs is the first step toward giving the AI full awareness of your browser — and it's already incredibly useful.
While there's a lot of hype around advanced MCP integrations (project tracking, build systems, etc.), debugging browser errors with MCP is the most accessible and practical place to start.
In this guide, you'll learn how to set it up using Cursor IDE, the most popular AI coding assistant today. The same approach works with tools like Cline or Windsurf if you're using one of those instead.
The Plan
This tutorial uses the Agent Desk AI plugin. While their docs are sufficient, they cover a lot of ground unnecessary to a simple setup. They're also slightly out of sync with the current version of Cursor, and glossed over some important concepts such as the difference between SSE and function MCPs.
We want the simplest possible proof-of-concept that lets us verify the AI can see and fix a browser error. So given that, the plan is:
- Create a new NextJS app
- Throw a simple error that will show in the browser logs
- Install the BrowserDesk tool
- Wire Cursor together to the MCP tool
- Verify the AI agent can now see the console errors and fix the issue
Stay Updated
Subscribe to my Substack newsletter for updates on AI tools and workflows for engineers building in the age of LLMs.
Creating The App
For this experiment we will just create a generic NextJS app:
npx create-next-app@latest mcp-test
and now we can just create a simple error in the main index file:
useEffect(() => {
// Simulate a bug
const broken = undefined;
// console.log(broken.length); // This will throw an error - Commented out to fix
}, []);
Now we have a nice big fat red error:
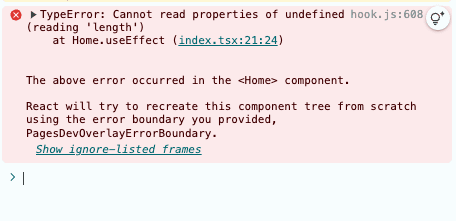
If we use Cursor to fix the error, it will tell us it has no way of seeing the error.
Installing and Configuring the AgentDeck BrowserTools tool
The BrowserTools plugin has 3 parts:
- A chrome extension that provides the information about the browser logs
- A Node server that knows how to talk to the chrome extension
- Another CLI tool that knows how to query the Node server for the browser information
MCP Function vs Server
This is already a few different moving parts, but what makes this especially confusing is that you're running a server but it's not AN MCP server.
An MCP server, in the traditional sense, exposes a manifest and an SSE endpoint that AI IDEs like Cursor can query directly. In this setup, we're using an MCP function, which Cursor runs as a local command. That function connects to a Node server, but that server isn't part of the MCP interface itself — it just gathers browser data.
Installing the Chrome Extension
Download the chrome extension here, then go to Chrome -> Extensions -> Load Unpacked and select the zip file. Make sure its running in Chrome.
Installing the Server
The server step is the simplest, as you just run:
npx @agentdeskai/browser-tools-server@latest`
in your terminal. Note that this should be done before running the browser-tools-mcp@latest
in the next step, despite the GitHub README mistakenly switching the order at one point.
Configure the MCP
The final step is to give Cursor the ability to run the command to talk to the server. To do that, we just go to Cursor->Settings->Install New MCP server and drop off this JSON:
{
"mcpServers": {
"browser-tools": {
"command": "npx",
"args": ["-y", "@agentdeskai/browser-tools-mcp@1.2.0"]
}
}
}
as you can see the MCP in this case is not a server but instead a CLI command.
It Should Work
You should see Cursor show that the MCP is now loaded:
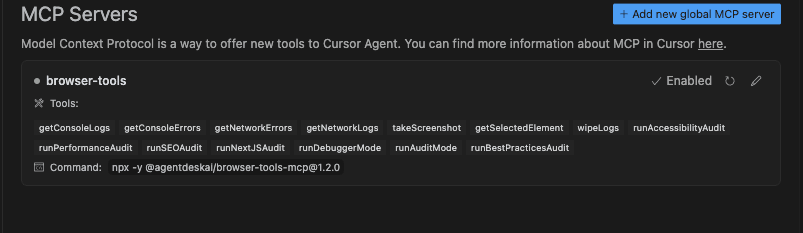
And now if you ask a model in Cursor if it sees the error, it should tell you it sees the error and be able to fix it.
Conclusion
Browser dev logs are a no-brainer use case for MCP and the first anyone working in webdev should setup. Hit me up on twitter @bill_prin or email waprin@gmail.com with any comments.